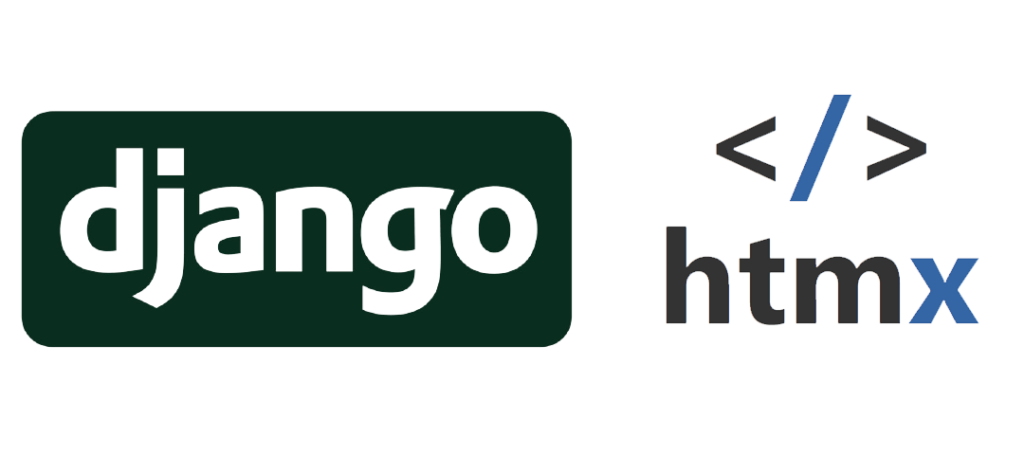
I gave a talk last night at the Auckland chapter meetup of Python New Zealand. The subject was the use of htmx with Django .
The JavaScript library htmx allows ‘native app like’ user experience for a Django project. Instead of refreshing the entire page, only the parts of the screen that actually need updating are refreshed. This eliminates those pesky flashes and reloads you get with conventional page refreshes. The result? A much smoother interface..
Now, normally, achieving this level of interactivity would require a full-fledged JavaScript-based frontend, along with the corresponding deep dive into React, Vue, or whichever framework is trending this month. But with htmx, you can get similar results by making relatively minor changes to your existing Django templates and views. No new framework, no need to sell your soul to JavaScript (at least not entirely) and you do away with large amounts of code serializing and de-serializing data.
In a Django context this is all made easier with the help of the django-htmx add-on (written by Adam Johnson). It provides the tools you need to integrate htmx into your Django project. In my presentation, I shared an overview of htmx and how combining it with django-htmx can deliver a smooth, engaging user experience—without the need to build a full-on JavaScript frontend or expose a mountain of API endpoints to service it.
That said, I’m not suggesting htmx is a silver bullet. There are plenty of scenarios where a “proper” JavaScript framework—be it React/Next.js, Vue, or Svelte—makes more sense. But I’m excited to explore more about where htmx fits into the Django ecosystem and how it can simplify things in the right contexts
Here are my slides from last night.